Mastering Stream Processing in Java: A Comprehensive Guide
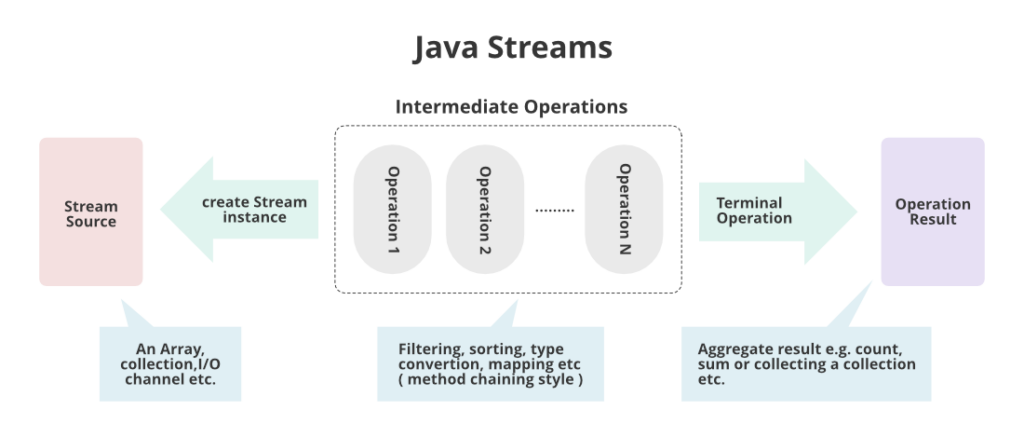
Introduction: In the world of Java programming, working with streams has become an essential skill for developers seeking to manipulate, transform, and process data efficiently. Java Streams, introduced in Java 8, provide a powerful and expressive API for working with collections of data in a functional style. In this comprehensive guide, we’ll explore the fundamentals of working with streams in Java, from the basics of stream creation and operation chaining to advanced techniques for parallel processing and stream optimization. Whether you’re new to Java Streams or looking to deepen your understanding, this guide will equip you with the knowledge and tools to become a proficient stream processing developer.
- Understanding Streams: Streams in Java represent a sequence of elements that support various operations to perform computations on those elements. Unlike collections, which are eagerly evaluated and stored in memory, streams are lazily evaluated, meaning elements are processed on-demand as operations are applied to them. This lazy evaluation allows for efficient processing of large datasets and enables developers to write concise and expressive code using functional programming paradigms.
- Creating Streams: There are several ways to create streams in Java, including from collections, arrays, files, and even from individual values. The
stream()
method, introduced in theCollection
interface, allows you to create a sequential stream from a collection. Similarly, theArrays.stream()
method enables you to create a stream from an array. Additionally, you can use theStream.of()
method to create a stream from individual values or theFiles.lines()
method to create a stream from the lines of a file. - Stream Operations: Once you have created a stream, you can perform a wide range of operations on it to manipulate, filter, and transform its elements. Stream operations are divided into two categories: intermediate operations and terminal operations. Intermediate operations, such as
filter()
,map()
, andflatMap()
, modify or transform the elements of the stream and return a new stream. Terminal operations, such asforEach()
,collect()
, andreduce()
, consume the elements of the stream and produce a result or side-effect. - Chaining Operations: One of the key features of Java Streams is the ability to chain multiple operations together to form a pipeline. This allows you to express complex data processing workflows in a concise and readable manner. For example, you can filter a stream to select only certain elements, map each element to a new value, and then collect the results into a new collection. By chaining operations together, you can create powerful and expressive data processing pipelines that efficiently manipulate streams of data.
- Filtering and Mapping: Filtering and mapping are two common operations performed on streams to select and transform elements based on certain criteria. The
filter()
operation allows you to select elements that satisfy a given predicate, while themap()
operation enables you to transform each element of the stream using a given function. Additionally, theflatMap()
operation can be used to flatten nested streams or transform each element into zero or more elements. - Reducing: Reducing is a terminal operation that aggregates the elements of a stream into a single result. The most common form of reduction is performed with the
reduce()
method, which takes an initial value and a binary operator and recursively combines the elements of the stream. For example, you can use thereduce()
operation to calculate the sum of the elements in a stream or find the maximum or minimum element. - Collecting: Collecting is another terminal operation that accumulates the elements of a stream into a collection or other data structure. The
collect()
method takes aCollector
as an argument, which specifies how the elements should be collected. Common collectors includetoList()
,toSet()
, andtoMap()
, which collect the elements into lists, sets, and maps, respectively. Additionally, you can create custom collectors using theCollector
interface to perform more complex collecting operations. - Parallel Stream Processing: Java Streams support parallel processing, allowing you to take advantage of multi-core processors to speed up data processing tasks. Parallel streams divide the elements of a stream into multiple chunks and process them concurrently on different threads. To create a parallel stream, simply call the
parallel()
method on the stream. However, it’s important to note that not all stream operations are suitable for parallel processing, and care must be taken to ensure thread safety and avoid race conditions. - Stream Optimization: To ensure optimal performance when working with streams, it’s important to understand the factors that can impact stream performance and how to optimize your code accordingly. Common optimization techniques include minimizing the number of intermediate operations, avoiding unnecessary boxing and unboxing of primitive values, and using parallel streams judiciously. Additionally, you can optimize stream performance by reducing the size of the dataset, optimizing resource usage, and leveraging specialized stream implementations for specific use cases.
- Conclusion: In conclusion, mastering stream processing in Java is a valuable skill for developers seeking to efficiently manipulate and process data in their applications. By understanding the fundamentals of stream creation, operation chaining, and parallel processing, you can leverage the power of Java Streams to write concise, expressive, and performant code. Whether you’re processing large datasets, implementing data transformations, or building complex data processing pipelines, Java Streams provide a versatile and powerful toolset for tackling a wide range of data processing tasks. So dive into the world of Java Streams, experiment with different operations and techniques, and unlock the full potential of stream processing in your Java applications.