How to Utilize Lisp and Scripting in BricsCAD
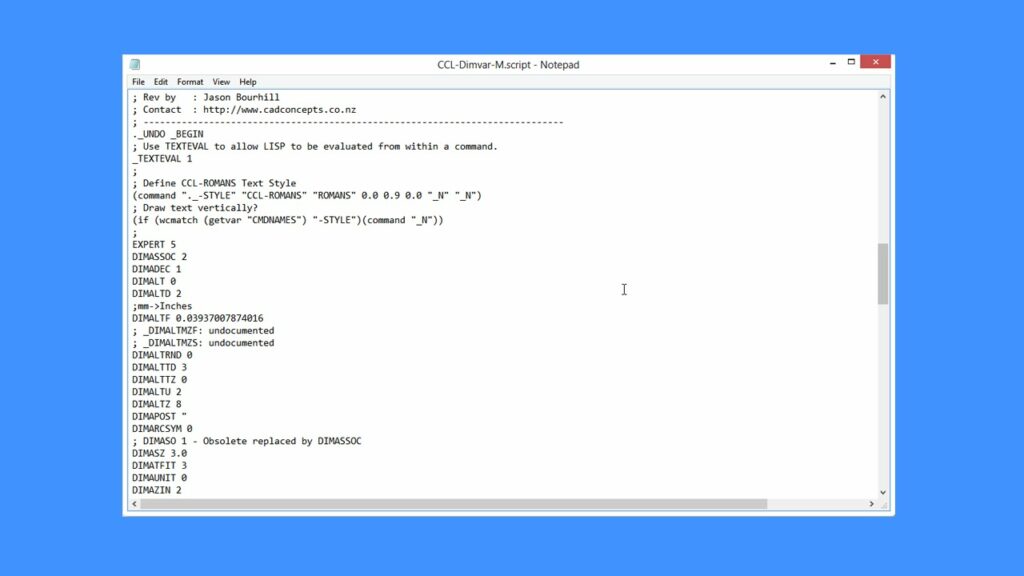
Introduction
BricsCAD, a popular CAD software, offers extensive customization capabilities through Lisp programming and scripting. Lisp, a powerful programming language, allows users to automate tasks, create custom commands, and enhance productivity within BricsCAD. This article will guide you through the fundamentals of using Lisp and scripting in BricsCAD, from setting up your environment to creating complex automation scripts.
Getting Started with Lisp in BricsCAD
1. Understanding Lisp Basics
Lisp stands for “LISt Processing” and is known for its simple syntax and powerful capabilities in automation and customization. BricsCAD supports AutoLISP, a dialect of Lisp tailored for AutoCAD-based applications.
2. Setting Up BricsCAD for Lisp Development
- Enable AutoLISP: Ensure AutoLISP support is enabled in BricsCAD. You can check this in the settings or preferences menu.
- Text Editor: Choose a text editor that supports Lisp syntax highlighting for writing and editing your Lisp scripts. Popular choices include Emacs, Notepad++, or even BricsCAD’s built-in editor.
3. Writing Your First Lisp Program
Let’s create a simple Lisp program that draws a line in BricsCAD:
(defun c:drawline ()
(command "line" pause pause)
)
- Explanation:
DEFUN
: Defines a new Lisp function namedDRAWLINE
.C:
prefix: Registers the function as a command callable from the command line in BricsCAD.COMMAND
: Executes a BricsCAD command, in this case, “LINE” followed by “PAUSE PAUSE” prompts.
4. Loading and Running Lisp Programs
- Loading: Use the
APPLOAD
command in BricsCAD to load your Lisp files. - Running: Once loaded, type the command name (e.g.,
DRAWLINE
) in the BricsCAD command line to execute your Lisp function.
Advanced Lisp Techniques
1. Parameter Handling
Lisp functions can accept parameters to customize behavior. For example:
(defun c:drawrectangle (/ width height)
(setq width (getreal "Enter width: "))
(setq height (getreal "Enter height: "))
(command "rectang" pause pause width height)
)
- Explanation:
GETREAL
: Prompts the user to enter a real number (e.g., width and height).RECTANG
: Draws a rectangle with specified width and height.
2. Error Handling
Implement error handling to improve script robustness:
(defun c:drawcircle ()
(if (setq center (getpoint "Center point: "))
(command "circle" center (getreal "Radius: "))
(prompt "Error: Invalid center point.")
)
)
- Explanation:
GETPOINT
: Prompts the user to pick a point.IF
: Conditional statement to check ifGETPOINT
returns a valid point.
Scripting in BricsCAD
Apart from Lisp, BricsCAD also supports scripting using languages like VBScript, JavaScript, and Python. Scripting allows for more complex automation and integration with external systems.
1. Using VBScript in BricsCAD
VBScript is a popular choice for scripting within BricsCAD due to its ease of use and Windows integration.
Sub DrawLine()
ThisDrawing.ModelSpace.AddLine _
ThisDrawing.Utility.GetPoint(, "Specify start point: "), _
ThisDrawing.Utility.GetPoint(, "Specify end point: ")
End Sub
- Explanation:
SUB
: Defines a subroutine namedDRAWLINE
.THISDRAWING
: Refers to the current drawing in BricsCAD.MODELSPACE.ADDLINE
: Adds a line to the model space.
2. Integrating Python Scripts
Python scripting in BricsCAD leverages the powerful Python ecosystem for CAD automation:
import bricscaddef draw_circle():
center = bricscad.Point(0, 0)
radius = bricscad.get_real("Enter radius: ")
bricscad.draw_circle(center, radius)
- Explanation:
BRICSCAD
: Python module providing access to BricsCAD API.DRAW_CIRCLE
: Function to draw a circle using user-defined center and radius.
Conclusion
Leveraging Lisp and scripting in BricsCAD empowers users to automate repetitive tasks, create custom commands, and integrate CAD workflows with other systems. Whether you’re a CAD designer looking to streamline your workflow or a developer extending BricsCAD’s functionality, mastering Lisp and scripting opens up a world of possibilities in CAD customization.
In this article, we’ve covered the basics of Lisp programming in BricsCAD, from setup and simple scripts to advanced techniques and scripting with other languages. With practice and exploration, you can harness the full potential of Lisp and scripting to make BricsCAD work more efficiently for your specific needs.
Start exploring and transforming your CAD workflows with Lisp and scripting in BricsCAD today!