Mastering Objective-C Programming for iOS Engineering Applications: A Comprehensive Guide
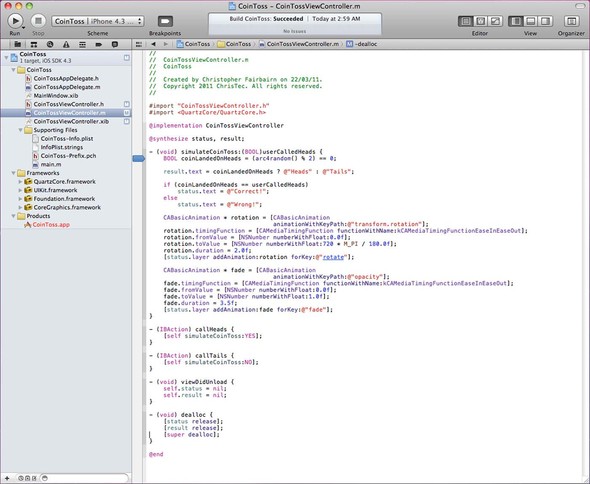
Introduction: Objective-C is a powerful programming language used for developing iOS and macOS applications. With its rich syntax, object-oriented features, and seamless integration with Apple’s frameworks, Objective-C has been the primary language for iOS app development for many years. In this comprehensive guide, we will delve into the principles, methodologies, and best practices of programming in Objective-C for iOS engineering applications. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge and skills to create robust, efficient, and user-friendly iOS applications using Objective-C.
Section 1: Introduction to Objective-C Programming
1.1 Overview of Objective-C: Objective-C is a superset of the C programming language that adds object-oriented capabilities and dynamic runtime features. Developed by Brad Cox and Tom Love in the early 1980s, Objective-C became popular for macOS and iOS development after Apple adopted it as the primary language for Cocoa and Cocoa Touch frameworks.
1.2 Key Features and Concepts: Explore the key features and concepts of Objective-C, including classes, objects, methods, properties, protocols, categories, and memory management. Understand how Objective-C combines the syntax of C with Smalltalk-style messaging and dynamic typing to provide a flexible and expressive programming environment for iOS app development.
Section 2: Setting Up Your Development Environment
2.1 Installing Xcode: Xcode is Apple’s integrated development environment (IDE) for macOS and iOS app development. Learn how to download and install Xcode from the Mac App Store and set up your development environment for Objective-C programming.
2.2 Creating a New Project: Create a new iOS project in Xcode using the Objective-C template. Choose the appropriate project settings, such as the project name, organization identifier, deployment target, and device compatibility, to configure your project for iOS app development.
Section 3: Understanding Objective-C Syntax and Language Constructs
3.1 Declaring Classes and Objects: Learn how to declare classes and create objects in Objective-C using the @interface and @implementation directives. Define instance variables, properties, and methods within class interfaces and implementations to encapsulate data and behavior in your iOS applications.
3.2 Working with Methods and Messages: Understand the concept of methods and messages in Objective-C and how they enable object-oriented programming. Define instance methods and class methods to perform operations on objects and send messages to invoke behavior in your iOS app.
3.3 Managing Memory: Explore memory management in Objective-C using manual reference counting (MRC) and automatic reference counting (ARC) techniques. Learn how to allocate and deallocate memory for objects, retain and release object references, and avoid memory leaks and retain cycles in your iOS applications.
Section 4: Interacting with iOS Frameworks
4.1 Using Foundation Framework: The Foundation framework provides essential classes and utilities for iOS app development. Discover how to use Foundation classes, such as NSString, NSArray, NSDictionary, and NSNumber, to manipulate strings, collections, dates, and other fundamental data types in your Objective-C code.
4.2 Accessing UIKit Framework: The UIKit framework provides user interface components and controls for iOS app development. Learn how to use UIKit classes, such as UIViewController, UIView, UIButton, UILabel, and UITableView, to create interactive and visually appealing user interfaces for your iOS applications.
4.3 Handling User Input and Events: Implement event handling and user interaction in your iOS applications using Objective-C and UIKit. Capture user input from touch events, gestures, and UI controls, and respond to user actions by updating the user interface, processing data, and triggering application logic in your Objective-C code.
Section 5: Advanced Topics in Objective-C Programming
5.1 Working with Blocks and Grand Central Dispatch (GCD): Explore blocks, a language feature introduced in Objective-C 2.0, and how they enable the creation of inline anonymous functions for asynchronous and concurrent programming tasks. Learn how to use blocks with Grand Central Dispatch (GCD) to perform background tasks, manage concurrency, and improve the responsiveness of your iOS applications.
5.2 Implementing Networking and Data Persistence: Integrate networking functionality and data persistence in your iOS applications using Objective-C and Apple’s frameworks. Learn how to make network requests, fetch and parse data from web services, and store data locally using APIs such as NSURLSession, NSURLConnection, and CoreData.
5.3 Enhancing App Performance and Optimization: Optimize the performance of your iOS applications using Objective-C techniques and best practices. Explore strategies for improving app responsiveness, reducing memory usage, minimizing CPU and battery consumption, and optimizing code execution to deliver a smooth and efficient user experience on iOS devices.
Section 6: Testing, Debugging, and Deployment
6.1 Testing Your App: Test your iOS applications using Xcode’s built-in testing and debugging tools. Learn how to run unit tests, UI tests, and performance tests to ensure the quality, reliability, and functionality of your Objective-C code.
6.2 Debugging and Troubleshooting: Debug and troubleshoot issues in your iOS applications using Xcode’s debugging features. Identify and fix runtime errors, exceptions, crashes, and memory leaks by inspecting variables, setting breakpoints, and stepping through code in the debugger.
6.3 Deploying Your App: Prepare your iOS application for distribution on the App Store or for deployment to test devices. Generate provisioning profiles, code signing certificates, and app identifiers, and configure app settings, icons, and metadata in Xcode before submitting your app for review and publication on the App Store.
Conclusion: Programming in Objective-C for iOS engineering applications offers developers a powerful and flexible platform for building sophisticated and feature-rich mobile apps. By mastering the principles, methodologies, and best practices outlined in this guide, developers can leverage Objective-C’s rich syntax, object-oriented features, and seamless integration with Apple’s frameworks to create robust, efficient, and user-friendly iOS applications. With its comprehensive set of tools, libraries, and APIs, Objective-C empowers developers to unleash their creativity, innovate new solutions, and deliver compelling experiences that delight users on iOS devices around the world.