Mastering Concurrency in Go: A Comprehensive Guide
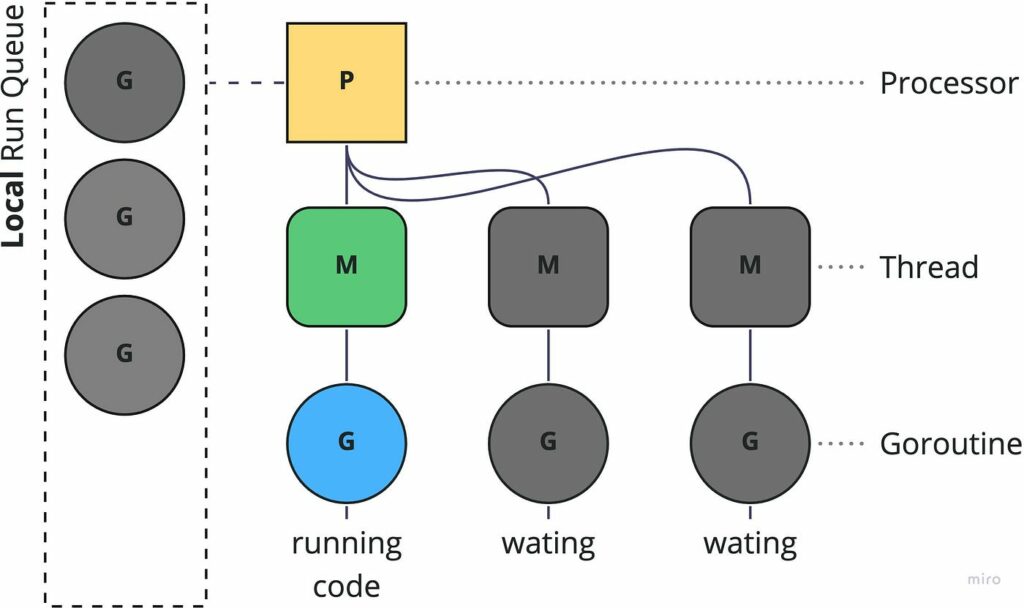
Introduction: Concurrency is a fundamental aspect of modern software development, enabling applications to perform multiple tasks concurrently and utilize available resources efficiently. In Go (or Golang), concurrency is a first-class citizen, with built-in language features and libraries designed to support concurrent programming. In this comprehensive guide, we’ll explore the principles of concurrency in Go, from goroutines and channels to synchronization primitives and patterns. Whether you’re new to Go or looking to deepen your understanding of concurrency, this guide will equip you with the knowledge and tools to effectively handle concurrent tasks and build robust, scalable applications.
- Understanding Concurrency in Go: Concurrency in Go revolves around the concept of goroutines, lightweight threads of execution that enable concurrent execution of code. Goroutines are managed by the Go runtime and are multiplexed onto operating system threads, allowing thousands or even millions of goroutines to run concurrently. Goroutines communicate with each other through channels, which serve as conduits for passing data between concurrent tasks. This combination of goroutines and channels forms the foundation of concurrent programming in Go.
- Goroutines: Goroutines are functions or methods that are executed concurrently with other goroutines within the same Go program. You can create a new goroutine by prefixing a function call with the
go
keyword, which instructs the Go runtime to execute the function in a separate goroutine. Goroutines are lightweight and have minimal overhead, making it easy to spawn thousands of them within a single Go program. By leveraging goroutines, you can perform concurrent tasks such as parallel computation, asynchronous I/O, and background processing with ease. - Channels: Channels are the primary means of communication and synchronization between goroutines in Go. A channel is a typed conduit through which you can send and receive values between goroutines. Channels can be created using the
make()
function with thechan
keyword followed by the type of values that the channel can transmit. You can send values to a channel using the<-
operator and receive values from a channel using the same operator in the opposite direction. Channels provide a powerful mechanism for coordinating concurrent tasks and sharing data between goroutines safely and efficiently. - Buffered and Unbuffered Channels: Channels in Go can be either buffered or unbuffered, depending on whether they have a fixed capacity for storing values. Unbuffered channels have a capacity of zero and require both a sender and a receiver to be ready before a value can be transmitted. Buffered channels, on the other hand, have a specified capacity and allow values to be stored temporarily until they are received by a goroutine. Buffered channels provide a way to decouple the sender and receiver, allowing them to operate asynchronously.
- Synchronization Primitives: In addition to channels, Go provides a variety of synchronization primitives for coordinating access to shared resources and ensuring safe concurrent execution. These primitives include mutexes, locks, condition variables, and atomic operations. Mutexes and locks provide exclusive access to shared data structures, preventing multiple goroutines from accessing them simultaneously. Condition variables enable goroutines to wait for specific conditions to be met before proceeding with execution. Atomic operations provide low-level synchronization primitives for performing atomic read-modify-write operations on shared variables.
- Patterns for Concurrent Programming: There are several common patterns and idioms for concurrent programming in Go that help ensure safe and efficient execution of concurrent tasks. These patterns include the producer-consumer pattern, worker pool pattern, fan-in/fan-out pattern, and select statement pattern. The producer-consumer pattern involves one or more goroutines producing data and sending it to a shared channel, while one or more consumer goroutines receive and process the data. The worker pool pattern involves creating a pool of worker goroutines that process tasks from a shared queue. The fan-in/fan-out pattern involves distributing work across multiple goroutines and aggregating the results. The select statement pattern allows goroutines to wait on multiple channels simultaneously and proceed with execution when data becomes available on any of the channels.
- Error Handling in Concurrent Code: Error handling in concurrent code presents unique challenges due to the asynchronous nature of goroutines and channels. It’s important to handle errors gracefully and propagate them to the appropriate goroutine for handling. One common approach is to use selective receive operations with channels to handle errors returned by concurrent tasks. Another approach is to use deferred functions to ensure that resources are properly cleaned up in the event of an error. Additionally, Go’s
context
package provides a mechanism for canceling and timing out concurrent tasks, which can help prevent resource leaks and improve the reliability of concurrent code. - Testing Concurrent Code: Testing concurrent code in Go presents its own set of challenges, as race conditions and timing issues can be difficult to reproduce and debug. Go’s built-in testing framework provides support for testing concurrent code using the
go test
command and thetesting
package. You can use techniques such as synchronization primitives, timeouts, and test fixtures to ensure deterministic behavior and avoid flakiness in your tests. Additionally, tools such as thego race
detector andgo vet
can help identify data races and other concurrency-related issues in your code. - Best Practices for Concurrent Programming: When writing concurrent code in Go, it’s important to follow best practices to ensure correctness, efficiency, and maintainability. Some best practices include minimizing shared mutable state, favoring composition over inheritance, using channels for communication and synchronization, avoiding premature optimization, and testing thoroughly for race conditions and concurrency bugs. By adhering to these best practices, you can write concurrent code that is robust, reliable, and easy to understand.
- Conclusion: In conclusion, mastering concurrency in Go is essential for building scalable, efficient, and responsive applications. By understanding the principles of goroutines, channels, synchronization primitives, and concurrent programming patterns, you can harness the full power of Go’s concurrency model to create high-performance, concurrent software systems. Whether you’re building web servers, distributed systems, or concurrent data processing pipelines, Go provides the tools and abstractions you need to handle concurrency effectively. So dive into the world of concurrent programming in Go, experiment with different techniques and patterns, and unlock the full potential of concurrent programming in your Go applications.